The Complete Data Structures and Algorithms Course in Java
Language: English | Size:4.9 GB
Genre:eLearning
Files Included :
1 Introduction.mp4 (22.6 MB)
MP4
1 What & Why is AVL Tree.mp4 (16.93 MB)
MP4
10 1 AVLTree.zip (1.93 KB)
ZIP
2 CreateAVLTree & Search Operation.mp4 (14.92 MB)
MP4
3 Left-Left Condition (Insertion).mp4 (103.56 MB)
MP4
4 Details of Rotation & Condition.mp4 (32.04 MB)
MP4
5 Left-Right Condition (Insertion).mp4 (33.05 MB)
MP4
6 Right-Right Condition (Insertion).mp4 (34.31 MB)
MP4
7 Right-Left Condition (Insertion).mp4 (34.32 MB)
MP4
8 Delete Node from AVL Tree (LL, LR, RL, RR Condition).mp4 (55.59 MB)
MP4
9 Delete AVL Tree.mp4 (5.78 MB)
MP4
1 What & Why is Trie.mp4 (12.33 MB)
MP4
2 Representation of Trie Data Structure.mp4 (11.08 MB)
MP4
3 Create Trie & Insert Word.mp4 (23.44 MB)
MP4
4 Prefix Search.mp4 (19.17 MB)
MP4
5 Search Word.mp4 (24.21 MB)
MP4
6 Delete Word.mp4 (56.94 MB)
MP4
7 1 Trie.zip (1.04 KB)
ZIP
1 Linear Search Algorithm.mp4 (11.23 MB)
MP4
2 1 LinearSearch.zip (385 B)
ZIP
3 Binary Search Algorithm.mp4 (24.83 MB)
MP4
4 1 BinarySearch.zip (446 B)
ZIP
1 Bubble Sort.mp4 (43.15 MB)
MP4
1 1 BubbleSort.zip (508 B)
ZIP
2 Selection Sort.mp4 (36.74 MB)
MP4
2 1 SelectionSort.zip (496 B)
ZIP
3 Insertion Sort.mp4 (48.52 MB)
MP4
3 1 InsertionSort.zip (425 B)
ZIP
4 Bucket Sort.mp4 (43.96 MB)
MP4
4 1 BucketSort.zip (784 B)
ZIP
5 Merge Sort.mp4 (65.69 MB)
MP4
5 1 MergeSort.zip (603 B)
ZIP
6 Quick Sort.mp4 (72.99 MB)
MP4
6 1 QuickSort.zip (365 B)
ZIP
7 Heap Sort.mp4 (27.18 MB)
MP4
7 1 HeapSort.zip (1.08 KB)
ZIP
1 What is recursion & Why we should use recursion.mp4 (29.08 MB)
MP4
2 Format of recursion.mp4 (6.15 MB)
MP4
3 How recursion works internally.mp4 (17.63 MB)
MP4
3 1 Factorial.zip (310 B)
ZIP
4 Nth Fibonacci.mp4 (18.78 MB)
MP4
4 1 Fib.zip (281 B)
ZIP
5 Recursion vs Iteration.mp4 (12 MB)
MP4
1 What & Why is Hashing.mp4 (25.07 MB)
MP4
2 Hashing Terminologies.mp4 (12.63 MB)
MP4
3 Hash Function.mp4 (27.03 MB)
MP4
4 Collision and Collison Resolution Technique.mp4 (53.61 MB)
MP4
5 Pros & Cons of Collisions Resolution Technique.mp4 (4.01 MB)
MP4
6 What if hashing is full.mp4 (9.06 MB)
MP4
7 How HashMap Works Internally.mp4 (52.6 MB)
MP4
1 What is Dynamic Programming & Why.mp4 (41.69 MB)
MP4
2 Top-Down Approach.mp4 (42.67 MB)
MP4
3 Bottom Up Approach.mp4 (11.4 MB)
MP4
4 Longest Increasing Subsequence.mp4 (36.45 MB)
MP4
5 House Robber.mp4 (41.41 MB)
MP4
6 Min Path Sum.mp4 (34.53 MB)
MP4
7 Longest Common Subsequence.mp4 (46.47 MB)
MP4
8 01 Knapsack Problem.mp4 (58.79 MB)
MP4
9 Regular Expression Matching.mp4 (94.89 MB)
MP4
1 What is Graph Data Structure.mp4 (36.87 MB)
MP4
10 BFS & DFS - Graph Traversal Techniques Explained.mp4 (71.08 MB)
MP4
11 BFS Algorithm (Breadth First Search).mp4 (22.04 MB)
MP4
11 1 BFS -- Algorithm.zip (881 B)
ZIP
12 DFS Algorithm (Depth First Search).mp4 (18.67 MB)
MP4
12 1 DFS -- Algorithm.zip (783 B)
ZIP
13 Toplogical Sort Explained.mp4 (47.74 MB)
MP4
14 Topological Sort Algorithm.mp4 (77.74 MB)
MP4
14 1 TopSortAlgorithm--Example-01.zip (1014 B)
ZIP
14 2 TopSortAlgorithm--Example-02.zip (1 KB)
ZIP
15 What is SSSP Problem (Single Source Shortest Path).mp4 (17.28 MB)
MP4
16 Why BFS & DFS Doesn't Works for SSSP Problem.mp4 (10.22 MB)
MP4
17 Dijkstra Algorithm for SSSP Problem.mp4 (80.85 MB)
MP4
17 1 DijkstraAlgorithm.zip (921 B)
ZIP
18 Why Dijstra Fail for Negative Cycle.mp4 (15.15 MB)
MP4
19 What is Edge Relaxation.mp4 (14.42 MB)
MP4
2 Graph Terminologies.mp4 (33.79 MB)
MP4
20 Dijsktra VS Bellman Ford's Algorithm for SSSP Problem.mp4 (23.29 MB)
MP4
21 Bellman Ford's Algorithm & Nagative Cycle Detection for SSSP Problem.mp4 (71.41 MB)
MP4
21 1 BellmanFord'sAlgorithm.zip (1.19 KB)
ZIP
22 What is All Pair Shortest Path Problem (APSP Problem).mp4 (9.45 MB)
MP4
24 Dijkstra & Bellman Ford's Algorithm for APSP Problem.mp4 (10.89 MB)
MP4
25 Floyd Warshall Algorithm Dynamic Programming.mp4 (122.24 MB)
MP4
26 What is Minimum Spanning Tree.mp4 (12.74 MB)
MP4
27 Prim's Algorithm -- Minimum Spanning Tree (MST).mp4 (82.42 MB)
MP4
28 Disjoint Set Data Structure.mp4 (99.53 MB)
MP4
29 What is Path Compression in Disjoint Set Data Structure.mp4 (15.58 MB)
MP4
3 Types of Graph.mp4 (12.83 MB)
MP4
30 Kruskal's Algorithm -- Minimum Spanning Tree (MST).mp4 (152.93 MB)
MP4
4 Graph Representation Technique.mp4 (9.11 MB)
MP4
5 When to use which representation.mp4 (23.77 MB)
MP4
6 Graph Representation using Adjacency Matrix (Undirected Graph).mp4 (109.28 MB)
MP4
6 1 UnweightedUndirectedUsingAdjacencyMatrix.zip (652 B)
ZIP
6 2 WeightedUndirectedUsingAdjacencyMatrix.zip (684 B)
ZIP
7 Graph Representation using Adjacency Matrix (Directed Graph).mp4 (64.78 MB)
MP4
7 1 UnweightedDirectedGraphAdjacencyMatrixRepresentation.zip (534 B)
ZIP
7 2 WeightedDirectedGraphAdjacencyMatrixRepresentation.zip (546 B)
ZIP
8 Graph Representation using Adjacency List (Unweighted Graph).mp4 (53.76 MB)
MP4
8 1 UnweightedDirectedGraphUsingAdjacencyList.zip (552 B)
ZIP
8 2 UnweightedUndirectedGraphUsingAdjacencyList.zip (561 B)
ZIP
9 Graph Representation using Adjacency List (Weighted Graph).mp4 (46.51 MB)
MP4
9 1 WeightedDirectedGraphUsingAdjacencyList.zip (671 B)
ZIP
9 2 WeightedUndirectedGraphUsingAdjacencyList.zip (674 B)
ZIP
1 What is Algorithm Runtime Analysis.mp4 (32.64 MB)
MP4
2 Why should we learn algorithm run time analysis.mp4 (43.18 MB)
MP4
3 Time Complexity Analysis Example #1.mp4 (17.06 MB)
MP4
4 Time Complexity Analysis Example #2.mp4 (19.24 MB)
MP4
1 What is an array & why we need an array.mp4 (18.43 MB)
MP4
10 Traverse 2D Array.mp4 (17.6 MB)
MP4
10 1 Traverse2DArray.zip (10.4 KB)
ZIP
11 1D Array Problem Move Zeroes.mp4 (13.14 MB)
MP4
12 1D Array Problem Remove Duplicates from Sorted Array.mp4 (30.66 MB)
MP4
12 1 RemoveDuplicates.zip (476 B)
ZIP
13 2D Array Problem Rotate Image.mp4 (34.96 MB)
MP4
13 1 RotateImage.zip (867 B)
ZIP
14 2D Array Problem Spiral Matrix.mp4 (63.83 MB)
MP4
14 1 SpiralMatrix.zip (10.71 KB)
ZIP
2 Types of array.mp4 (19.05 MB)
MP4
3 How is an array represented in RAM.mp4 (11.88 MB)
MP4
4 Create a 1D Array.mp4 (9.76 MB)
MP4
5 Traverse 1D Array.mp4 (6.23 MB)
MP4
6 Get, Insert, Update & Delete Operations in 1D Array.mp4 (14.11 MB)
MP4
6 1 OperationsIn1DArray.zip (514 B)
ZIP
7 Searching Algorithms (Linear Search + Binary Search).mp4 (30.81 MB)
MP4
7 1 BinarySearchAlgorithm.zip (461 B)
ZIP
7 2 LinearSearchAlgorithm.zip (419 B)
ZIP
8 Create a 2D Array.mp4 (14.24 MB)
MP4
9 Get, Insert & Update Operations in 2D Array.mp4 (11.79 MB)
MP4
9 1 OperationsIn2DArray.zip (574 B)
ZIP
1 What is Linked List.mp4 (14.81 MB)
MP4
10 Insertion in CSLL (Circular Singly Linked List).mp4 (23.96 MB)
MP4
11 Deleting Node & Deleting Entire Linked List (Circular Singly Linked List).mp4 (15.11 MB)
MP4
12 1 MyCircularSinglyLinkedList.zip (1.09 KB)
ZIP
13 Creation & Insertion Operations in DLL (Doubly Linked List).mp4 (9.97 MB)
MP4
14 Traversing & Searching in DLL (Doubly Linked List).mp4 (15.02 MB)
MP4
15 Insertion in DLL (Doubly LInked List).mp4 (12.52 MB)
MP4
16 Deleting Node & Deleting Enire Linked List (Doubly Linked List).mp4 (13.71 MB)
MP4
17 1 MyDoublyLinkedList.zip (1.09 KB)
ZIP
18 Creation & Insertion Operations in CDLL (Circular Doubly Linked LIst).mp4 (12.89 MB)
MP4
19 Traversing & Searching in CDLL (Circular Doubly Linked List).mp4 (9.08 MB)
MP4
2 Different Types of Linked Lists.mp4 (13.63 MB)
MP4
20 Insertion in CDLL (Circular Doubly Linked List).mp4 (18.56 MB)
MP4
21 Deleting Node & Deleting Entire Linked List (Circular Doubly Linked List).mp4 (24.46 MB)
MP4
22 1 MyCircularDoublyLinkedList.zip (1.1 KB)
ZIP
23 Linked List Problem Reverse a Singly Linked List -- Recursive Solution.mp4 (30.5 MB)
MP4
24 Linked List Problem Reverse a Singly Linked List -- Iterative Solution.mp4 (26.48 MB)
MP4
3 Creation & Insertion Operations in SLL (Singly Linked List).mp4 (16.62 MB)
MP4
3 1 MySinglyLinkedList(Creation&Insertion).zip (703 B)
ZIP
4 Insertion (At Index, At Begining & At End) Operations in SSL(Singly LInked List).mp4 (29.84 MB)
MP4
4 1 MySinglyLinkedList.zip (970 B)
ZIP
4 2 MySinglyLinkedList(Insertion).zip (970 B)
ZIP
5 Travering & Searching in SLL (Singly Linked List).mp4 (10.04 MB)
MP4
5 1 MySinglyLinkedList(TraversingSearching).zip (1.09 KB)
ZIP
6 Deleting Node & Deleting Entire Linked List (Singly Linked List).mp4 (18.95 MB)
MP4
6 1 MySinglyLinkedList(DeletingNode&EntireLinkedList).zip (1.24 KB)
ZIP
7 1 MySinglyLinkedList.zip (1.24 KB)
ZIP
8 Creation & Insertion Operations in CSLL (Circular Singly Linked List).mp4 (16.02 MB)
MP4
9 Traversing & Searching in CSLL (Circular Singly Linked List).mp4 (13.41 MB)
MP4
1 What is Stack.mp4 (26.96 MB)
MP4
10 Stack Problem Decode String.mp4 (48.84 MB)
MP4
2 Stack Implementation Options.mp4 (5.69 MB)
MP4
3 Array - Create, Push & Pop.mp4 (26.81 MB)
MP4
4 Array - Peek, isEmpty, isFull & deleteStack.mp4 (8.97 MB)
MP4
5 1 StackUsingArray.zip (664 B)
ZIP
6 Linked List - Create, Push & Pop.mp4 (12.91 MB)
MP4
7 Linked List - Peek, isEmpty, & deleteStack.mp4 (7.56 MB)
MP4
8 1 StackUsingLinkedList.zip (689 B)
ZIP
9 Stack Problem Valid Parentheses.mp4 (19.1 MB)
MP4
1 What is Queue.mp4 (15 MB)
MP4
2 Queue Implementation Options.mp4 (2.96 MB)
MP4
3 Array - Linear Queue.mp4 (16.88 MB)
MP4
4 1 LinearQueueUsingArray.zip (805 B)
ZIP
5 Array - Circular Queue.mp4 (33.2 MB)
MP4
6 1 CircularQueueUsingArray.zip (748 B)
ZIP
7 Linked List - Linear Queue.mp4 (21.13 MB)
MP4
8 1 LinearQueueUsingLinkedList.zip (681 B)
ZIP
1 What is Tree & Why should we learn tree.mp4 (12.69 MB)
MP4
10 Delete Binary Tree.mp4 (5.9 MB)
MP4
11 Tree Traversal Techniques Inorder, Preorder & Postorder Traversal.mp4 (125.83 MB)
MP4
12 Preorder Traversal Details Recursive + Iterative.mp4 (98.91 MB)
MP4
13 Inorder Traversal Details Recursive + Iterative.mp4 (103.87 MB)
MP4
14 Postorder Traversal Details Recursive + Iterative.mp4 (112.29 MB)
MP4
15 Level Order Traversal Details Recursive + Iterative.mp4 (68.39 MB)
MP4
16 1 BinaryTreeUsingLinkedList.zip (1.86 KB)
ZIP
17 Create Binary Tree & Insert Value Array Implementation.mp4 (22.83 MB)
MP4
18 Search for a Value in Binary Tree.mp4 (10.55 MB)
MP4
19 Delete Node from Binary Tree.mp4 (16.91 MB)
MP4
2 Tree Terminologies Root, Leaf, Edge, Ancestor, Depth of Tree.mp4 (20.67 MB)
MP4
20 Delete Binary Tree.mp4 (5.75 MB)
MP4
21 Preorder Traversal.mp4 (13.43 MB)
MP4
22 Inorder Traversal.mp4 (12.48 MB)
MP4
23 Postorder Traversal.mp4 (15.59 MB)
MP4
24 Level order Traversal.mp4 (6.85 MB)
MP4
25 1 BinaryTreeUsingArray.zip (11.11 KB)
ZIP
3 Tree Terminologies Predecessor & Successor.mp4 (16.88 MB)
MP4
4 What is Binary Tree.mp4 (14.11 MB)
MP4
5 Types of Binary Tree.mp4 (23.27 MB)
MP4
6 How is Tree Represented Linked List & Array Representation.mp4 (19.37 MB)
MP4
7 Create Binary Tree & Insert Value Linked List Implementation.mp4 (38.54 MB)
MP4
8 Search for a Value in Binary Tree.mp4 (15.35 MB)
MP4
9 Delete Value from Binary Tree.mp4 (61.97 MB)
MP4
1 What & Why is BST.mp4 (17.28 MB)
MP4
2 Creation & Insertion in BST.mp4 (24.78 MB)
MP4
3 Traversing BST.mp4 (15.08 MB)
MP4
4 Searching in BST.mp4 (20.82 MB)
MP4
5 Deletion Node in BST.mp4 (43.1 MB)
MP4
6 Deletion of BST.mp4 (5.2 MB)
MP4
7 1 BST.zip (1.08 KB)
ZIP
1 What is Binary Heap & Why.mp4 (11.6 MB)
MP4
2 Heap Implementation Options.mp4 (12.06 MB)
MP4
3 Insert into Binary Heap.mp4 (38.29 MB)
MP4
4 Size, IsEmpty & Peek Operation.mp4 (7.93 MB)
MP4
5 Extract Operation.mp4 (60.13 MB)
MP4
6 Delete Heap.mp4 (5.23 MB)
MP4
7 1 HeapUsingArray.zip (1.14 KB)
ZIP
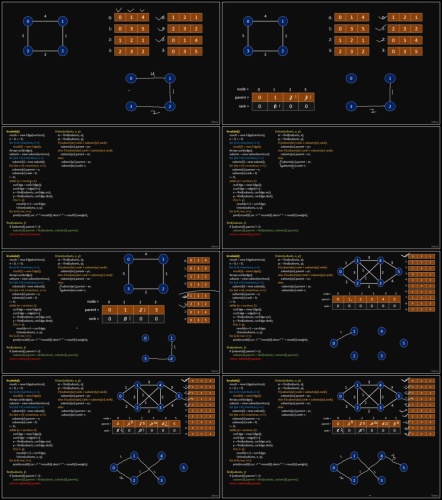