Algorithms-and-Data-Structures-in-Python-INTERVIEW-QA
Language: English | Size:4.13 GB
Genre:eLearning
Files Included :
1 Introduction.mp4 (18.02 MB)
MP4
1 What are queues.mp4 (10.54 MB)
MP4
2 Queue implementation.mp4 (25.37 MB)
MP4
1 Max in a stack problem solution.mp4 (30.52 MB)
MP4
2 Queue with stack problem solution.mp4 (23.52 MB)
MP4
3 Queue with stack problem solution - recursion.mp4 (24.68 MB)
MP4
1 What are binary search trees.mp4 (41.5 MB)
MP4
10 Binary Search Tree implementation III.mp4 (71.82 MB)
MP4
11 Practical (real-world) applications of trees.mp4 (12.83 MB)
MP4
2 Binary search trees theory - search, insert.mp4 (13.19 MB)
MP4
3 Binary search trees theory - delete.mp4 (12.73 MB)
MP4
4 Binary search trees theory - in-order traversal.mp4 (14.39 MB)
MP4
5 Binary search trees theory - running times.mp4 (7.4 MB)
MP4
6 Binary search tree implementation I.mp4 (25.09 MB)
MP4
7 Binary Search Tree implementation II.mp4 (35.41 MB)
MP4
8 Stack memory visualization - finding max (min) items.mp4 (11.79 MB)
MP4
9 Stack memory visualization - tree traversal.mp4 (17.15 MB)
MP4
1 Compare binary trees solution.mp4 (35.59 MB)
MP4
1 Motivation behind balanced binary search trees.mp4 (8.19 MB)
MP4
10 AVL tree implementation V.mp4 (7.07 MB)
MP4
11 Practical (real-world) applications of balanced binary search trees.mp4 (15.45 MB)
MP4
2 What are AVL trees.mp4 (16.36 MB)
MP4
3 AVL trees introduction - height.mp4 (38.94 MB)
MP4
4 AVL trees introduction - rotations.mp4 (22.49 MB)
MP4
5 AVL trees introduction - illustration.mp4 (9.11 MB)
MP4
6 AVL tree implementation I.mp4 (27.64 MB)
MP4
7 AVL tree implementation II.mp4 (41.86 MB)
MP4
8 AVL tree implementation III.mp4 (96.54 MB)
MP4
9 AVL tree implementation IV.mp4 (129.99 MB)
MP4
1 What are red-black trees.mp4 (29.66 MB)
MP4
2 The logic behind red-black trees.mp4 (20.45 MB)
MP4
3 Red-black trees - recoloring and rotation cases.mp4 (21.03 MB)
MP4
4 Red-black tree illustrations.mp4 (12.74 MB)
MP4
5 Red-black tree implementation I.mp4 (18.46 MB)
MP4
6 Red-black tree implementation II.mp4 (15.85 MB)
MP4
7 Red-black tree implementation III.mp4 (73.47 MB)
MP4
8 Red-black tree implementation IV.mp4 (16.25 MB)
MP4
9 Differences between red-black tree and AVL trees.mp4 (7.86 MB)
MP4
1 What are priority queues.mp4 (12.81 MB)
MP4
10 Heap implementation III.mp4 (38.29 MB)
MP4
11 Heaps in Python.mp4 (11.94 MB)
MP4
2 Heap introduction - basics.mp4 (29.21 MB)
MP4
3 Heap introduction - array representation.mp4 (18.86 MB)
MP4
4 Heap introduction - remove operation.mp4 (17.96 MB)
MP4
5 Using heap data structure to sort (heapsort).mp4 (12.32 MB)
MP4
6 Heap introduction - operations complexities.mp4 (39.86 MB)
MP4
7 Binomial and Fibonacci heaps.mp4 (17.98 MB)
MP4
8 Heap implementation I.mp4 (18.17 MB)
MP4
9 Heap implementation II.mp4 (75.95 MB)
MP4
1 Interview question #1 - solution.mp4 (20.26 MB)
MP4
2 Interview question #2 - solution.mp4 (27.72 MB)
MP4
1 What are associative arrays.mp4 (17.99 MB)
MP4
2 Hashtable introduction - basics.mp4 (42.34 MB)
MP4
3 Hashtable introduction - collisions.mp4 (48.53 MB)
MP4
4 Hashtable introduction - dynamic resizing.mp4 (34.03 MB)
MP4
5 Linear probing implementation I.mp4 (24.62 MB)
MP4
6 Linear probing implementation II.mp4 (55.58 MB)
MP4
7 Linear probing implementation III.mp4 (12.6 MB)
MP4
8 Dictionaires in Python.mp4 (11.59 MB)
MP4
9 Practical (real-world) applications of hashing.mp4 (20.91 MB)
MP4
1 Graph theory overview.mp4 (12.74 MB)
MP4
2 Adjacency matrix and adjacency list.mp4 (18.98 MB)
MP4
3 Applications of graphs.mp4 (12.99 MB)
MP4
1 Why to use data structures.mp4 (22.35 MB)
MP4
2 Data structures and abstract data types.mp4 (14.19 MB)
MP4
1 Breadth-first search introduction.mp4 (37.73 MB)
MP4
2 Breadth-first search implementation.mp4 (25.66 MB)
MP4
3 What are WebCrawlers (core of search engines).mp4 (31.38 MB)
MP4
4 WebCrawler basic implementation.mp4 (43.17 MB)
MP4
5 Depth-first search introduction.mp4 (31.24 MB)
MP4
6 Depth-first search implementation.mp4 (22.69 MB)
MP4
7 Memory management BFS vs DFS.mp4 (29.76 MB)
MP4
1 Interview question #1 - solution.mp4 (9.82 MB)
MP4
2 Depth-first search and stack memory visualisation.mp4 (13.67 MB)
MP4
3 Interview question #2 - solution.mp4 (79.46 MB)
MP4
1 What is the shortest path problem.mp4 (14.2 MB)
MP4
2 Dijkstra algorithm visualization.mp4 (28.43 MB)
MP4
3 Dijkstra algorithm implementation I - Edge, Node.mp4 (38.1 MB)
MP4
4 Dijkstra algorithm implementation II - algorithm.mp4 (73.77 MB)
MP4
5 Dijkstra algorithm implementation III - testing.mp4 (36.45 MB)
MP4
6 Dijktsra's algorithm with adjacency matrix representation.mp4 (24.29 MB)
MP4
7 Adjacency matrix representation implementation.mp4 (78.91 MB)
MP4
8 Shortest path algorithms applications.mp4 (18.48 MB)
MP4
9 What is the critical path method (CPM).mp4 (13.7 MB)
MP4
1 What is the Bellman-Ford algorithm.mp4 (43.39 MB)
MP4
2 Bellman-Ford algorithm visualization.mp4 (10.7 MB)
MP4
3 Bellman-Ford algorithm implementation I - Node, Edge.mp4 (4.89 MB)
MP4
4 Bellman-Ford algorithm implementation II - the algorithm.mp4 (50.42 MB)
MP4
5 Bellman-Ford algorithm implementation III - testing.mp4 (19.56 MB)
MP4
6 Greedy algorithm or dynamic programming approach.mp4 (34.18 MB)
MP4
1 How to use Bellman-Ford algorithm on the FOREX.mp4 (16.5 MB)
MP4
2 Interview question #1 - solution.mp4 (20.84 MB)
MP4
1 What is the disjoint set data structure.mp4 (39.56 MB)
MP4
2 Disjoint sets visualization.mp4 (10.56 MB)
MP4
3 Kruskal's algorithm introduction.mp4 (55.13 MB)
MP4
4 Kruskal algorithm implementation I - basic classes.mp4 (19.71 MB)
MP4
5 Kruskal algorithm implementation II - disjoint set.mp4 (62.66 MB)
MP4
6 Kruskal algorithm implementation III - algorithm.mp4 (22.75 MB)
MP4
7 Kruskal algorithm implementation VI - testing.mp4 (16.2 MB)
MP4
1 What is the Prim-Jarnik algorithm.mp4 (25.59 MB)
MP4
2 Prims-Jarnik algorithm implementation I.mp4 (83.38 MB)
MP4
3 Prims-Jarnik algorithm implementation II.mp4 (31.64 MB)
MP4
4 Applications of spanning trees.mp4 (29.45 MB)
MP4
1 Sorting introduction.mp4 (25.75 MB)
MP4
10 Insertion sort introduction.mp4 (22.63 MB)
MP4
11 Insertion sort implementation.mp4 (15.35 MB)
MP4
12 Shell sort introduction.mp4 (11.95 MB)
MP4
13 Shell sort implementation.mp4 (15.03 MB)
MP4
14 Quicksort introduction.mp4 (34 MB)
MP4
15 Quicksort introduction - example.mp4 (41.3 MB)
MP4
16 Quicksort implementation.mp4 (38.21 MB)
MP4
17 What is the worst-case scenario for quicksort.mp4 (18.51 MB)
MP4
18 Merge sort introduction.mp4 (19.57 MB)
MP4
19 Merge sort implementation.mp4 (27.28 MB)
MP4
2 What is stability in sorting.mp4 (17.08 MB)
MP4
20 Stack memory and merge sort visualization.mp4 (18.56 MB)
MP4
21 Hybrid algorithms introduction.mp4 (19.11 MB)
MP4
22 Non-comparison based algorithms.mp4 (5.48 MB)
MP4
23 Counting sort introduction.mp4 (35.52 MB)
MP4
24 Counting sort implementation.mp4 (32.01 MB)
MP4
25 Radix sort introduction.mp4 (50.5 MB)
MP4
26 Radix sort implementation.mp4 (47.74 MB)
MP4
3 What is adaptive sorting.mp4 (19.42 MB)
MP4
4 Bogo sort introduction.mp4 (7.71 MB)
MP4
5 Bogo sort implementation.mp4 (46.55 MB)
MP4
6 Bubble sort introduction.mp4 (12.54 MB)
MP4
7 Bubble sort implementation.mp4 (14.71 MB)
MP4
8 Selection sort introduction.mp4 (30.08 MB)
MP4
9 Selection sort implementation.mp4 (14.23 MB)
MP4
1 Algorithms Visualization App.mp4 (7.44 MB)
MP4
1 What are array data structures.mp4 (25 MB)
MP4
2 Arrays introduction - operations.mp4 (24.95 MB)
MP4
3 Arrays in Python.mp4 (25.93 MB)
MP4
4 What are real arrays in Python.mp4 (16.84 MB)
MP4
1 Reversing an array in-place solution.mp4 (10.24 MB)
MP4
2 Palindrome problem solution.mp4 (23.81 MB)
MP4
3 Integer reversion problem solution.mp4 (22.2 MB)
MP4
4 Anagram problem solution.mp4 (17.95 MB)
MP4
5 Duplicates in an array problem solution.mp4 (31.55 MB)
MP4
1 What are linked lists.mp4 (19.08 MB)
MP4
2 Linked list introduction - operations.mp4 (33.41 MB)
MP4
3 Linked list implementation I.mp4 (27.5 MB)
MP4
4 Linked list implementation II.mp4 (14.46 MB)
MP4
5 Linked list implementation III.mp4 (29.86 MB)
MP4
6 Comparing linked lists and arrays.mp4 (14.42 MB)
MP4
7 Practical (real-world) applications of linked lists.mp4 (36.46 MB)
MP4
1 What are doubly linked lists.mp4 (29.5 MB)
MP4
2 Doubly linked list implementation.mp4 (39.49 MB)
MP4
3 Running time comparison linked lists and arrays.mp4 (15.09 MB)
MP4
1 Finding the middle node in a linked list solution.mp4 (18.9 MB)
MP4
2 Reverse a linked list in-place solution.mp4 (28.12 MB)
MP4
1 What are stacks.mp4 (10.57 MB)
MP4
2 Stacks in memory management (stacks and heaps ).mp4 (8.89 MB)
MP4
3 Stack memory visualization.mp4 (16.53 MB)
MP4
4 Stack implementation.mp4 (25.33 MB)
MP4
5 Practical (real-world) applications of stacks.mp4 (16.11 MB)
MP4
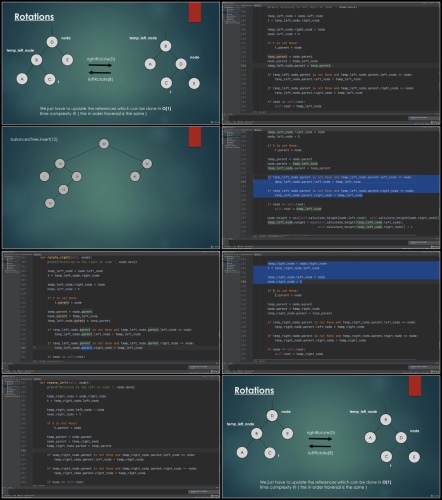
